This post will help you to integrate Salesforce with WordPress and access the blogs in Salesforce from WordPress. Here we are getting the blog from a linked WordPress account and displaying it in a lightning modal popup in Salesforce.
Steps to be implemented:
1. Create an app in WordPress application manager [create App]. Redirect URL for this application will be the lightning component application URL which was created to run the whole process. After the application is created we can get the OAuth information which includes client id and client secret.
Below images will show how to create Application in wordpress.
2. Add the following endpoints in remote site settings in salesforce org.
- https://public-api.wordpress.com/oauth2/authorize
- https://public-api.wordpress.com/oauth2/token
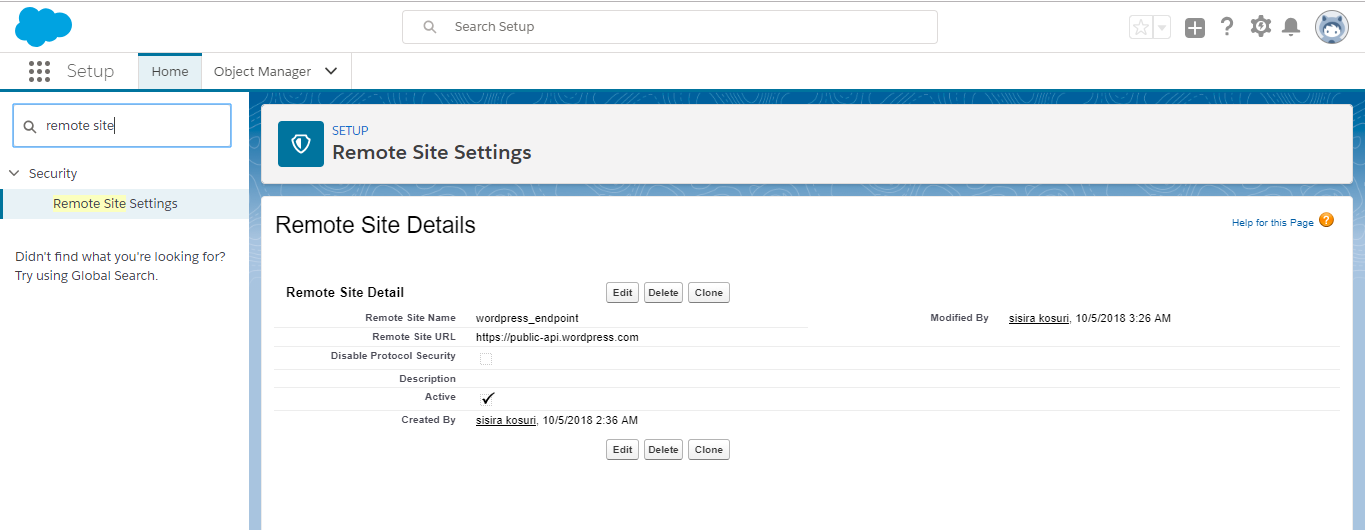
3. For better understanding I have a lightning component with three buttons which will authorize, get the access token and get the blogs from the wordpress.
Lightning Component:
[sourcecode language=”java”]
<aura:component controller=”accesstoken” implements=”force:appHostable, flexipage:availableForAllPageTypes, flexipage:availableForRecordHome, force:hasRecordId, forceCommunity:availableForAllPageTypes, force:lightningQuickAction” access=”global” >
<aura:attribute name=”url” type=”String” />
<aura:attribute name=”accesstoken” type=”String” />
<aura:attribute name=”content” type=”string” />
<aura:attribute name=”code” type=”string” />
<aura:handler name=”init” value=”{!this}” action=”{!c.doInit}”/>
<lightning:layout verticalAlign=”center” horizontalAlign=”center” >
<lightning:layoutitem padding=”around-small” >
<lightning:layout verticalAlign=”center” horizontalAlign=”center”>
<lightning:button label=”Authorize”
variant=”brand”
class=”slds-button slds-show”
onclick=”{!c.Authorize}”/>
<lightning:button label=”Request AccessToken”
variant=”brand”
class=”slds-button slds-show”
onclick=”{!c.GetAccess}”/>
<lightning:button label=”Get Blog”
variant=”brand”
class=”slds-button slds-show”
onclick=”{!c.send}”/>
</lightning:layout>
</lightning:layoutitem>
</lightning:layout>
<div class=”slds-hide” aura:id=”conmodal” style=”height: 1800px;”>
<section role=”dialog” tabindex=”-1″ aria-labelledby=”modal-heading-01″ aria-modal=”true” aria-describedby=”modal-content-id-1″ class=”slds-modal slds-fade-in-open”>
<div class=”slds-modal__container”>
<header class=”slds-modal__header” style=”background: #05668D;”>
<h2 id=”modal-heading-01″ class=”slds-text-heading_medium slds-hyphenate” style=”color:white;”><b>Blog</b></h2>
</header>
<div class=”slds-modal__content slds-p-around_medium” aura:id=”modal-content-id-1″>
<div class=”slds-container–center slds-container–small slds-m-top–small”>
<aura:unescapedHtml value=”{!v.content}”/>
</div>
</div>
<footer class=”slds-modal__footer slds-show” aura:id=”footer1″>
<button class=”slds-button button1″ aura:id=”button-modal-1″ style=”background: #05668D;color:white;width:130px;” onclick=”{!c.hideModal}”>
Close
</button>
</footer>
</div>
</section>
<div class=”slds-backdrop slds-backdrop_open”></div>
</div>
</aura:component>
[/sourcecode]
Below image is our lightning app with the three buttons.

4. On click of Authorize button we are hitting the wordpress endpoint for authorization.
Client side controller:
[sourcecode language=”java”]
Authorize: function(component, event, helper){
window.location.replace(‘https://public-api.wordpress.com/oauth2/authorize?’+’client_id=$clientid& amp; amp; redirect_uri=$redirecturl& amp; amp; response_type=code&amp;scope=global’);
}
[/sourcecode]
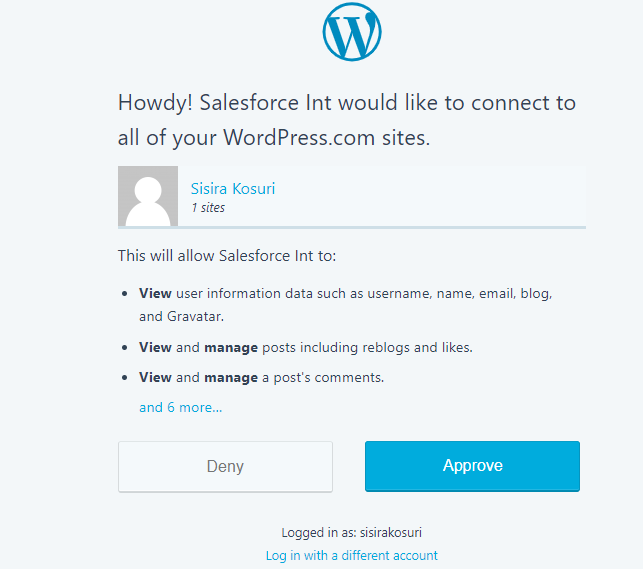
After approving we will be redirected to our lightning application. Now we need the Access Token to make API calls. For getting the access token we need the authorization code which we got in the redirected page URL.
5. Now click on the Request Access Token button.
Client side Controller:
[sourcecode language=”java”]
GetAccess: function(component, event, helper){
var action=component.get(“c.getaccess”);
action.setParams({‘code’:component.get(“v.code”)});
action.setCallback(this,function(response){
var state=response.getState();
if(state===’SUCCESS’)
{
var result=response.getReturnValue();
component.set(“v.accesstoken”,result);
}
});
$A.enqueueAction(action);
}
[/sourcecode]
Apex code:
[sourcecode language=”java”]
public static string getaccess(string code)
{
string clientid=$CLIENTID;
String redirect_uri = $APPURL;
String endPoint = ‘ https://public-api.wordpress.com/oauth2/token’;
string clientSecret=$CLIENTSECRET;
String requestTokenBody = ‘client_id=’+clientid+’&redirect_uri=’+redirect_uri+’&client_secret=’+
clientSecret+’&code=’+code+
‘&grant_type=authorization_code’;
Http http = new Http();
httpRequest httpReq = new httpRequest();
httpReq.setEndPoint(endPoint);
httpReq.setBody(requestTokenBody);
httpReq.setMethod(‘POST’);
HttpResponse response = new HttpResponse();
response = http.send(httpReq);
Map TokenInfo = (Map)JSON.deserializeUntyped(response.getBody());
string access=String.valueOf(TokenInfo.get(‘access_token’));
return access;
}
[/sourcecode]
6. Now in response we will get the access token. With the help of the access token we will get the blog details.
[sourcecode language=”java”]
send : function(component, event, helper) {
var action=component.get(“c.getBlogs”);
var access=component.get(“v.accesstoken”);
var url=”https://public-api.wordpress.com/rest/v1.1/me/posts”;
action.setParams({‘url’:url,’access’:access});
action.setCallback(this,function(response){
var state=response.getState();
if(state===’SUCCESS’)
{
var result=response.getReturnValue();
component.set(“v.content”,result);
}
});
$A.enqueueAction(action);
}
[/sourcecode]
The above example illustrate how to get the blog details. we are displaying the blog in the modal popup on click of Get Blog button.
[sourcecode language=”java”]
@auraenabled
public static string getBlogs(string url,string access)
{
Http http = new Http();
httpRequest httpReq = new httpRequest();
httpReq.setHeader(‘Authorization’,’Bearer ‘+access);
httpReq.setEndPoint(url);
httpReq.setMethod(‘GET’);
HttpResponse response = new HttpResponse();
response = http.send(httpReq);
Map lisdata=(Map)JSON.deserializeUntyped(String.valueOf(response.getBody()));
list values=(list)lisdata.get(‘posts’);
map smap=(Map)values[0];
string result=string.valueof(smap.get(‘content’));
return result;
}
[/sourcecode]
Displaying blog in Modal popup:
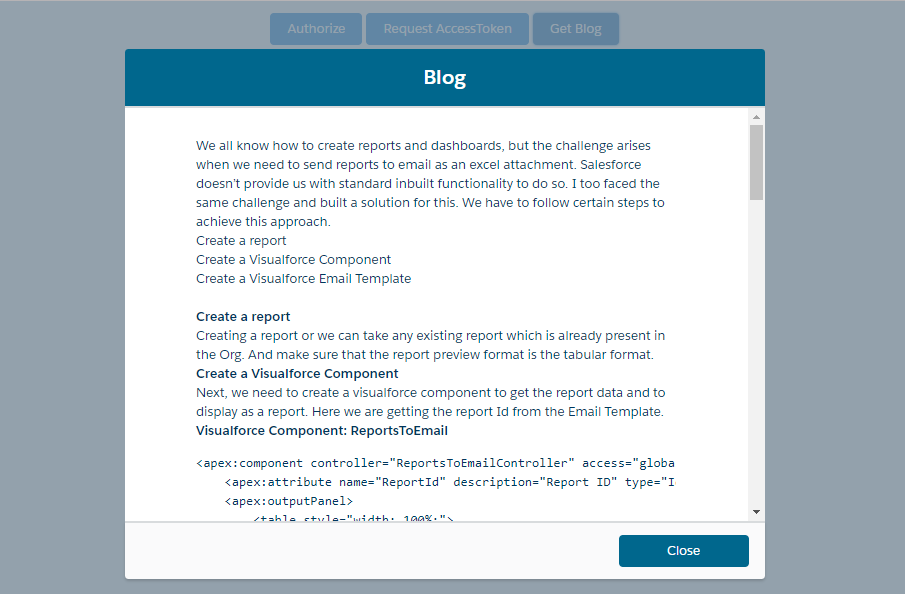
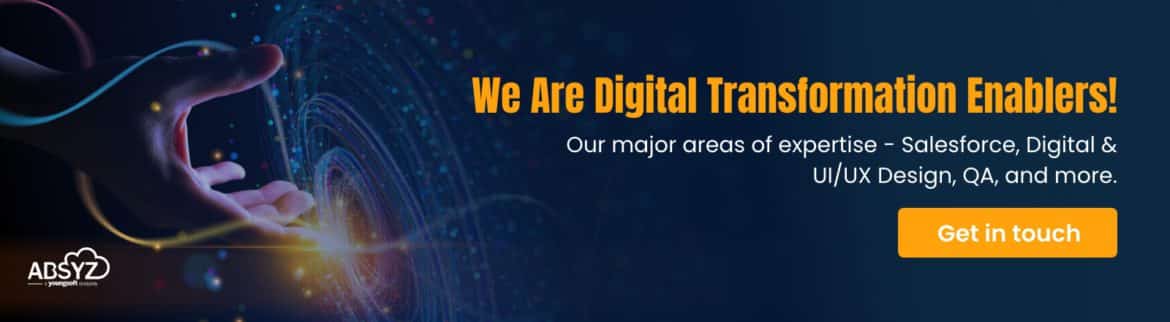