Everyone loves to use a custom component which can be placed in any object irrespective of whether its standard or custom. In this blog, I am going to introduce one such component, which is the reusable map component.
What it does?
By placing this component in your Lightning page, you can display the map based on the address field you choose. My component has two main parts:
- Picklist: This displays all the available address fields in your object. Be it standard or custom. Based on the value/field you choose from the picklist, a corresponding map will be displayed by taking the address stored in that field.(Note: Custom fields will be displayed provided they contain ‘Address’ in their field labels.)
- Map: This will display the address the user wishes to see on the map.
Sounds interesting!!! Let’s see how this can be achieved.
Prerequisites:
- Lightning enabled org
- Leaflet Javascript Library and CSS Stylesheet. Download from here.
- Google Map API key. Get your own here.
Now that we have all the necessary components, let’s dive into the code.
Steps:
- Upload the leaflet.zip file you downloaded before as a static resource in your org.
- Write an apex controller that displays the picklist values as well as processes the address value in the field. The code is as below:
[sourcecode language=”java”]
public class myMapApexController {
@auraenabled
public static List < String > getAddressFields(Id recsId) { //This method retrieves all the standard
//as well as custom address fields available in a particular object
List < String > options = new List < String > ();
Schema.SObjectType sobjectType = recsId.getSObjectType();
String sobjectName = sobjectType.getDescribe().getName();
List < String > availableFields = new List < String > ();
Map < String, Schema.SObjectField > objectFields = Schema.getGlobalDescribe().get(sobjectName).getDescribe().fields.getMap();
availableFields.add(‘–None–‘);
for (String s: objectFields.keySet()) {
Schema.DescribeFieldResult lfieldLabel = objectFields.get(s).getDescribe();
system.debug(‘LABEL::’ + lfieldLabel.getLabel());
Schema.DisplayType dType = lfieldLabel.getType();
string fieldType = String.ValueOf(dType);
system.debug(‘fieldType::’ + fieldType);
if (fieldType.equalsIgnoreCase(‘ADDRESS’)) //Checks for compound address fields
{
availableFields.add(lfieldLabel.getLabel().toUpperCase());
} else if (s.containsIgnoreCase(‘Address’) && s.endsWithIgnoreCase(‘__c’)) //Checks for custom field labels that have ‘Address’ in it
{
availableFields.add(lfieldLabel.getLabel().toUpperCase());
}
}
System.debug(‘availableFields::’ + availableFields);
return availableFields;
}
@auraenabled
public static String PopulateLatituteLongitude(Id recsId, String opt) { //This method queries the address from the record
//and returns the Lattitude and Longitude from the address
String city;
String street;
String state;
String code;
String country;
String addr;
String chosenValue;
string selFieldType;
Schema.SObjectType sobjectType = recsId.getSObjectType();
String sobjectName = sobjectType.getDescribe().getName();
Map < String, Schema.SObjectField > objectFields = Schema.getGlobalDescribe().get(sobjectName).getDescribe().fields.getMap();
for (String s: objectFields.keySet()) {
Schema.DescribeFieldResult lfieldLabel = objectFields.get(s).getDescribe();
Schema.DisplayType dType = lfieldLabel.getType();
string fieldType = String.ValueOf(dType);
if (lfieldLabel.getLabel().equalsIgnoreCase(opt)) {
chosenValue = s; //Get the API name of the value selected from picklist
selFieldType = fieldType;
}
}
String buildQuery = ‘SELECT id,’ + chosenValue + ‘ from ‘ + sobjectName + ‘ where id= ” + recsId + ”’;
System.debug(buildQuery);
sObject sObjRec = database.query(buildQuery);
if (selFieldType.equalsIgnoreCase(‘Address’)) {
Address compaddr = (Address) sObjrec.get(chosenValue);
street = compaddr.getStreet();
city = compaddr.getCity();
state = compaddr.getState();
code = compaddr.getPostalCode();
country = compaddr.getCountry();
addr = street + ‘+’ + city + ‘+’ + state + ‘+’ + code + ‘+’ + country;
} else {
addr = String.valueOf(sObjrec.get(chosenValue));
}
System.debug(‘Address::’ + addr);
string apiKey = ‘<span style=”color: #ff0000;”>USE YOUR OWN GOOGLE MAP API KEY HERE</span>’; //Unique alpha numeric key
//This is the key for server applications.
String modAddr = addr.replace(‘ ‘, ‘,’);
modAddr = modAddr.replace(‘-‘, ‘+’);
//modAddr = ‘Aditya+Enclave,Whitefields,Hitech+City,+Hyderabad’;
String url = ‘https://maps.googleapis.com/maps/api/geocode/xml?’;
url += ‘address=’ + modAddr;
url += ‘&key=’ + apiKey;
system.debug(url);
Http h = new Http();
HttpRequest req = new HttpRequest();
req.setHeader(‘Content-type’, ‘application/x-www-form-urlencoded’);
req.setHeader(‘Content-length’, ‘0’);
req.setEndpoint(url);
req.setMethod(‘POST’);
String responseBody = ”;
HttpResponse res = h.send(req);
responseBody = res.getBody();
///*Response body will include this… 46.8647086 -96.8262901 */
string geometryString = ”;
string locationString = ”;
string latitudeValue = ”;
string longitudeValue = ”;
Dom.Document doc = res.getBodyDocument();
Dom.XMLNode address = doc.getRootElement();
Dom.XMLNode result = address.getChildElement(‘result’, null);
Dom.XMLNode geometry = result.getChildElement(‘geometry’, null);
Dom.XMLNode location = geometry.getChildElement(‘location’, null);
latitudeValue = location.getChildElement(‘lat’, null).getText();
longitudeValue = location.getChildElement(‘lng’, null).getText();
return latitudeValue + ‘;’ + longitudeValue;
}
}
[/sourcecode]
The whole class is divided into two methods:
- getAddressFields(): This method takes the record id as parameter and returns all the address fields available in that object, which is then displayed as picklist values in the component.
- PopulateLatituteLongitude(): This method takes the record id and the value/field chosen from the picklist and builds the dynamic query as shown above. The address that we pass to the map should be of this format:- ‘Aditya+Enclave,Whitefields,Hitech+City,+Hyderabad’. Spaces replaced with ‘,’ and ‘-‘ replaced with ‘+’ characters. We are then making a REST API call to maps.googleapis.com and passing the API key as well as the address we generated. From the response, we get the Lattitude and Longitude which is then returned in ‘Lattitude;Longitude’ format.
3. Next, build a new lightning component as follows:
[sourcecode language=”java”]
<aura:component implements=”force:appHostable, flexipage:availableForAllPageTypes, flexipage:availableForRecordHome, force:hasRecordId, forceCommunity:availableForAllPageTypes” controller=”myMapApexController”>
<aura:attribute name=”recordId” type=”String” />
<aura:attribute name=”availvals” type=”String[]” />
<aura:handler name=”init” value=”{!this}” action=”{!c.doInit}” />
<ui:inputSelect aura:id=”getsel” class=”slds-input” change=”{!c.onSingleSelectChange}”>
<aura:iteration items=”{!v.availvals}” var=”val”>
<ui:inputSelectOption text=”{!val}” />
</aura:iteration>
</ui:inputSelect>
<ltng:require styles=”/resource/leaflet/leaflet.css” />
<ltng:require scripts=”/resource/leaflet/leaflet.js” />
<div aura:id=”mapnew” id=”mapnew” style=”height: 300px”>
<div align=”center”>
<h1 style=”font-size: 2em;”>SELECT A VALUE FROM PICKLIST</h1>
</div>
</div>
</aura:component>
[/sourcecode]
Every time we change the picklist value, the “onSingleSelectChange” method is called which indirectly calls the apex PopulateLatituteLongitude() and passes the currently selected picklist value.
4. The controller for the above component is as follows:
[sourcecode language=”java”]
({
doInit: function(component, event, helper) {
var lat;
var lng;
var recId = component.get(“v.recordId”);
console.log(recId);
var action = component.get(“c.getAddressFields”);
action.setParams({
“recsId”: recId
});
action.setCallback(this, function(res) {
var state = res.getState();
if (state === “SUCCESS”) {
console.log(state);
var str = res.getReturnValue();
component.set(‘v.availvals’, str);
}
});
$A.enqueueAction(action);
},
onSingleSelectChange: function(component, event, helper) {
var lat;
var lng;
var recId = component.get(“v.recordId”);
console.log(recId);
var map = null;
var leafl;
document.getElementById(‘mapnew’).innerHTML = “”;
document.getElementById(‘mapnew’).innerHTML = “
<div style=”height: 300px” class=”map” id=”map”></div>
“;
var selected = component.find(“getsel”).get(“v.value”);
var action = component.get(“c.PopulateLatituteLongitude”);
action.setParams({
“recsId”: recId,
“opt”: selected
});
action.setCallback(this, function(res) {
var state = res.getState();
if (state === “SUCCESS”) {
console.log(state);
var str = res.getReturnValue();
var arr = str.split(“;”);
lat = parseFloat(arr[0]);
lng = parseFloat(arr[1]);
setTimeout(function() {
map = new L.map(‘map’, {
zoomControl: true
});
map.setView(new L.LatLng(lat, lng), 16);
L.tileLayer(‘https://server.arcgisonline.com/ArcGIS/rest/services/World_Street_Map/MapServer/tile/{z}/{y}/{x}’, {
attribution: ‘Tiles © Esri’
}).addTo(map);
// Add marker
L.marker([lat, lng]).addTo(map)
.bindPopup(‘The Location’);
map.invalidateSize();
console.log(map);
});
}
});
$A.enqueueAction(action);
}
})
[/sourcecode]
Here we handle the response we receive from the controller. We extract the Lattitude and Longitude from the string and pass both values to the map function
[sourcecode language=”java”]
map = new L.map(‘map’, {
zoomControl: true
});
map.setView(new L.LatLng(lat, lng), 16);
L.tileLayer(‘https://server.arcgisonline.com/ArcGIS/rest/services/World_Street_Map/MapServer/tile/{z}/{y}/{x}’, {
attribution: ‘Tiles © Esri’
}).addTo(map);
// Add marker
L.marker([lat, lng]).addTo(map)
.bindPopup(‘The Location’);
[/sourcecode]
This part, creates the map and sets the map view to the passed Lattitude and Longitude values.
[sourcecode language=”java”]
document.getElementById(‘mapnew’).innerHTML = “”;
document.getElementById(‘mapnew’).innerHTML = “
<div style = “height: 300px” class=”map” id=”map”></div>
“;
[/sourcecode]
Whenever we change the picklist value, we are creating new map instance. Thus we need to remove the previously initialized dom element from the component and create a fresh one.
5. Next, to position the map, paste the below code in the CSS part of the component:
[sourcecode language=”java”]
.THIS.map {
position: relative;
top: 0;
left: 0;
right: 0;
bottom: 0;
}
[/sourcecode]
6. Lastly, place this component in your record detail page.
There you go! Your reusable map component is ready.
Note: This again is just the basic code. It can be optimized and customized according to one’s requirement.
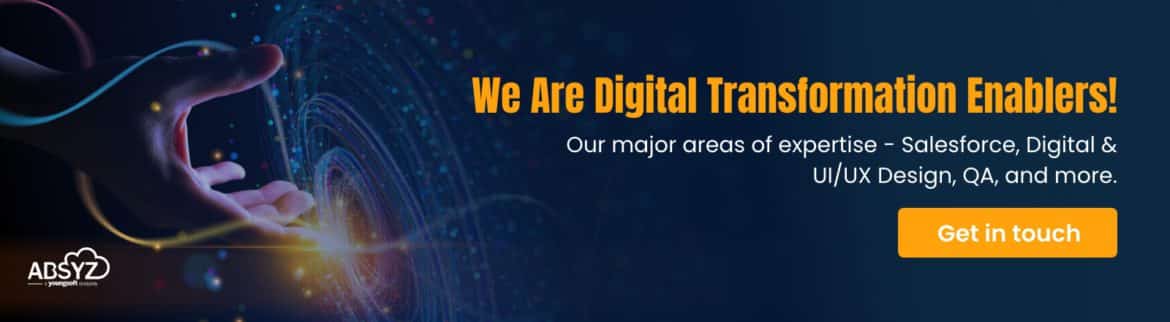