Posting message to social media through Salesforce makes it easy as Salesforce provide all flexibility in developing customer relationships. Posting and getting information from one of the social media helps to increase the number of customers and achieve targets.
Let suppose any company is having their LinkedIn page where users should log in and upload status or image. Instead, we can promote, improve sales, hire people and much more through Salesforce. Here we can upload and retrieve posts from LinkedIn to Salesforce using REST API. LinkedIn provides API calls for developers that can be referred in the documentation (https://developer.linkedin.com/docs). Developers can also test the callouts using LinkedIn REST Console.
First, create a LinkedIn account and proceed to create a company page as shown below.
Now to access LinkedIn we need to create an app. For that, you need to keep your account active.
click on My Apps to create an app
Fill in the details and submit.
After submitting go to Application settings -> Authorization where you will get the client key and client secret key. Provide permissions for the application and authorized redirect link to OAuth 2.0 as shown below.
To register the user go to the REST API console and click on the drop-down menu Authentication select OAuth 2.0. On clicking on it you are asked to redirect to user to access your basic LinkedIn profile information. Click on allow to access basic information. If you are unable to get the page then refer the link given (https://developer.linkedin.com/docs/oauth2). Enter the URL in the console to get access token. Currently, all access tokens are issued with a 60-day lifespan.
We have a different way of accessing the LinkedIn like to Sign In with LinkedIn, post on LinkedIn, Manage company pages and added details to Profiles. Here we are doing POST and GET method to an XXX company page. In this link, we have all the details how to manage our company page (https://developer.linkedin.com/docs/company-pages).
From Salesforce I provide status, link, and image fields to do a POST method to LinkedIn. The image is given in an URL format. When the button is clicked it gets posted to LinkedIn.
POST Method
First, add the link to remote site settings as specified in the code. Identify your company id in the URL of that page like this – https://www.linkedin.com/company/12345678/. Send these parameters through callout to LinkedIn as a JSON format. In the response, we get the status updated Id.
[sourcecode language=”java”]
public static void postRequest(String post,String link,String image){
String NewLine=’n’;
String NewLinereplace=’ ‘;
String newPost=post.replace(NewLine, NewLinereplace);
if(image!=null)
{
// pass the values in JSON format
String text = ‘{“visibility”: { “code”: “anyone” },”comment”: “‘+newPost+'”,”content”: {“submitted-url”: “‘+link+'”,”submitted-image-url”:”‘+image+'”}}’;
HttpRequest req = new HttpRequest();
//https://api.linkedin.com – add the link to remote site settings
//12345678 – give your company id
//oauth2_access_token – provide your access token
req.setEndpoint(‘https://api.linkedin.com/v1/companies/12345678/shares?format=json&oauth2_access_token=AQW…QQqA&format=json’);
req.setMethod(‘POST’);
req.setHeader(‘Content-Type’ , ‘application/json’);
req.setBody(text);
//Make request
Http http = new Http();
HTTPResponse res = http.send(req);
system.debug(‘response’+res.getBody());//in response we get posts id
}
else
{
//pass the values in JSON format
String text = ‘{“visibility”: { “code”: “anyone” },”comment”: “‘+newPost+'”,”content”: {“submitted-url”: “‘+link+'”}}’;
HttpRequest req = new HttpRequest();
//https://api.linkedin.com – add the link to remote site settings
//12345678 – give your company id
//oauth2_access_token – provide your access token
req.setEndpoint(‘https://api.linkedin.com/v1/companies/1234567/shares?format=json&oauth2_access_token=AQW…QQqA&format=json’);
req.setMethod(‘POST’);
req.setHeader(‘Content-Type’ , ‘application/json’);
req.setBody(text);
//Make request
Http http = new Http();
HTTPResponse res = http.send(req);
system.debug(‘response’+res.getBody());//in response we get posts id
}
}
[/sourcecode]
GET Method
To GET, we make an API call out to with company Id and access token
[sourcecode language=”java”]
public static void getRequest(){
HttpRequest req = new HttpRequest();
//https://api.linkedin.com – add the link to remote site settings
//12345678 – give your company id
//oauth2_access_token – provide your access token
req.setEndpoint(‘https://api.linkedin.com/v1/companies/12345678/updates?oauth2_access_token=AQW…QQqA&format=json’);
req.setMethod(‘GET’);
//Make request
Http http = new Http();
HTTPResponse res = http.send(req);
//resopnse body we get the information in JSON format containing id and message
system.debug(‘response’+res.getBody());
}
[/sourcecode]
From Salesforce
We get Status, Link, and Image URL from the user.
[sourcecode language=”java”]
<aura:component controller=”PosttoSocialMediaEXT” implements=”force:appHostable, flexipage:availableForAllPageTypes, flexipage:availableForRecordHome, force:hasRecordId, forceCommunity:availableForAllPageTypes, force:lightningQuickAction” access=”global”>
<aura:attribute name=”status” type=”String”/>
<aura:attribute name=”link” type=”String”/>
<aura:attribute name=”image” type=”String”/>
<aura:attribute name=”LinkedIn” type=”Boolean” default=”false”/>
<lightning:card title=”Social Media Post”>
<div class=”slds-align_absolute-center”>
<aura:set attribute=”title”>
<div style=”width:40%;”>
<div class=”slds-align_absolute-center”>
<p style=”font-family:serif;font-size: 40px;”>Social Media Post</p>
</div>
</div>
</aura:set>
<div style=”width:40%;”>
Post Message:
<lightning:textarea label=”” name=”myTextArea” value=”{!v.status}”
maxlength=”1000″ />
Post Link:
<lightning:textarea label=”” name=”myTextArea” value=”{!v.link}”
maxlength=”300″ />
Post Image Link:
<lightning:textarea label=”” name=”myTextArea” value=”{!v.image}”
maxlength=”300″ /><br/>
<div>
<lightning:input type=”checkbox” label=”Add To LinkedIn” name=”LinkedId” checked=”{!v.LinkedIn}” />
</div>
<div class=”slds-align_absolute-center”>
<lightning:button variant=”brand” label=”Submit” onclick=”{! c.handleClick }” />
</div>
</div>
</div>
</lightning:card>
</aura:component>
[/sourcecode]
From Salesforce, through javascript controller, we pass the values to apex methods.
[sourcecode language=”java”]
({
handleClick : function(component, event, helper) {
var action = component.get(“c.postStatus”);
action.setParams({ Post : component.get(“v.status”),
link : component.get(“v.link”),
image : component.get(“v.image”),
linkdIn : component.get(“v.LinkedIn”),
});
action.setCallback(this,function(response){
var State = response.getState();
if(State = ‘SUCCESS’){
var toastEvent = $A.get(“e.force:showToast”);
toastEvent.setParams({
“title”: “Success!”,
“message”: “The Status has been updated successfully.”
});
toastEvent.fire();
}
});
$A.enqueueAction(action);
}
})
[/sourcecode]
OUTPUT
User fill the details
The post on LinkedIn as shown below.
If you have any questions please feel free to post it in the comment.
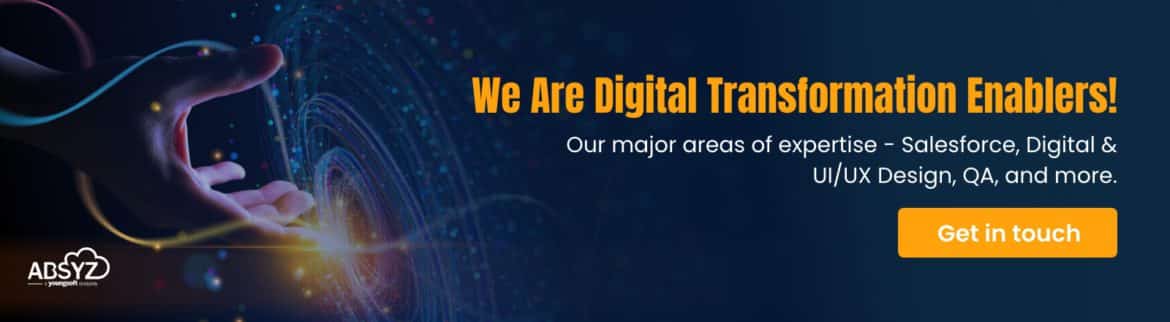