In salesforce we have several requirement to navigate to Account location or Lead
location on google map. Mostly sales representative need to navigate to exact
account location. In most of the case we look to integrate google map with salesforce
which could be costly and will have some maintenance charge as we need to buy
google API subscription. Google map navigation can be done without integrating
salesforce with google but we have to know account geolocation co-ordinate where we want to navigate.
To store the
Account geolocation co-ordinate, we need to create a custom field in account of
data type Geoloction. In this field we can store latitude and longitude of
account.
There is two ways of populating this field
- By directly populating the value in account record.
- We can create a tab on account record page which set current location co-ordinate as geolocation.
Second option is only applicable if we are at account location.
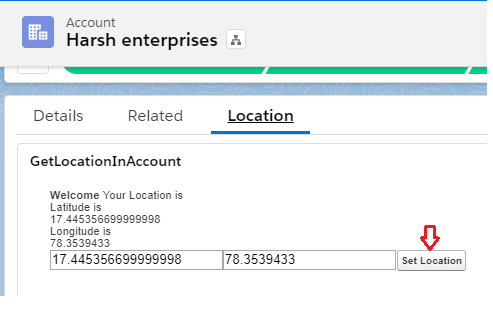
Click on set Location to update the account record with current geolocation co-ordinate.
Above Location tab has visualforce page linked to Location Tab.
Find Visualforce page code below.
<apex:page standardController="Account" extensions="GetLocationInAccountController" >
<!-- Begin Default Content REMOVE THIS -->
<apex:form id="form">
<h1>Welcome </h1> Your Location is<br/>
Latitude is
<div id="startLat">
</div>
Longitude is
<div id="startLon">
</div>
<!-- <apex:commandbutton style="border-radius:20px;background: #7acdf2;" action="{!getpresentinfo}" value="Get Location"/> -->
<!-- <apex:outputText value="The unformatted time right now is: {!dd}" /> -->
<!-- <apex:commandbutton style="border-radius:20px;background: #7acdf2;" onclick="ab(); return false;" value="checkin location"/> -->
<apex:inputText id="Latitude" value="{!Lat}" Label="Latitude"/>
<apex:inputText id="Longitude" value="{!Lon}" Label="Longitude"/>
<apex:commandbutton action="{!location}" value="Set Location"/>
</apex:form>
<script>
window.onload = function ab() {
var startPos;
var geoSuccess = function(position) {
startPos = position;
document.getElementById('startLat').innerHTML = startPos.coords.latitude;
document.getElementById('startLon').innerHTML = startPos.coords.longitude;
document.getElementById('{!$Component.form.Latitude}').value=startPos.coords.latitude;
document.getElementById('{!$Component.form.Longitude}').value=startPos.coords.longitude;
};
navigator.geolocation.getCurrentPosition(geoSuccess);
};
</script>
<script src="https://maps.google.com/maps/api/js?sensor=false"></script>
<script type="text/javascript">
var geocoder = new google.maps.Geocoder();
var latLng = new google.maps.LatLng(document.getElementById('{!$Component.form.Latitude}').value,
document.getElementById('{!$Component.form.Longitude}').value);
if (geocoder) {
geocoder.geocode({ 'latLng': latLng}, function (results, status) {
if (status == google.maps.GeocoderStatus.OK) {
console.log(results[0].formatted_address);
}
else {
console.log("Geocoding failed: " + status);
}
});
}
</script>
</apex:page>
Extension Code
public class GetLocationInAccountController
{
private ApexPages.StandardController standardController;
public Decimal Lat{get;set;}
public Decimal Lon{get;set;}
public GetLocationInAccountController(ApexPages.StandardController standardController)
{
this.standardController = standardController;
}
public PageReference location(){
ID recordid=standardController.getId();
System.debug('ID--'+recordid);
system.debug('Latitude---'+lat);
System.debug('Longitude-----'+lon);
list<Account> ss=new list<Account>();
for(Account s:[select id,name,Geolocation__Latitude__s,Geolocation__Longitude__s from Account where id=:recordid]){
s.Geolocation__Latitude__s=Lat;
s.Geolocation__Longitude__s=lon;
ss.add(s);
}
update ss;
PageReference pageRef = new PageReference('/'+recordid);
return pageRef.setRedirect(true);
}
}
Once we have account record with geolocation we can create a lightning component which will have account name and buttons to navigate to google map from current location to account location and buttons to know the distance between current location to account location.
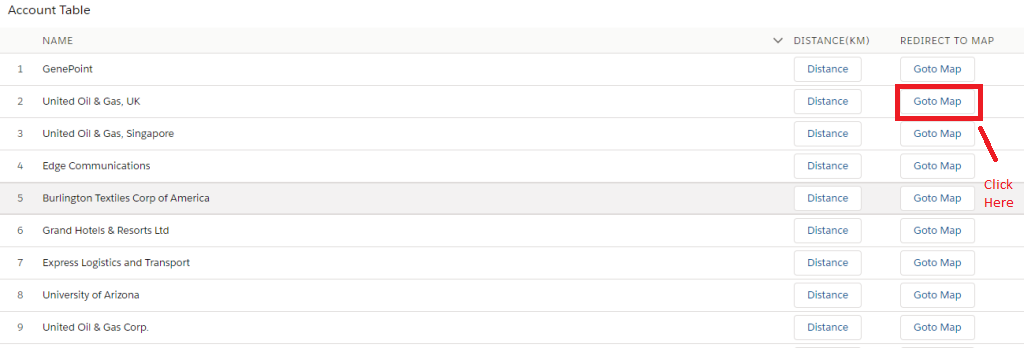
Click on Goto Map button to navigate to google map.
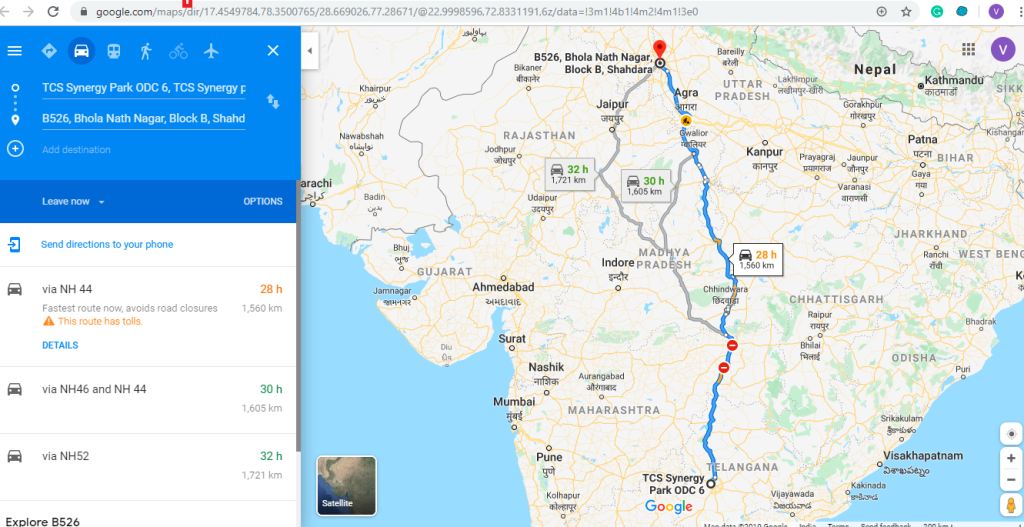
Code for the component is given below.
Component:
<aura:component controller="GoogleMapNavigationController" implements="force:appHostable, flexipage:availableForAllPageTypes, flexipage:availableForRecordHome, force:hasRecordId, forceCommunity:availableForAllPageTypes, force:lightningQuickAction" access="global" >
<aura:attribute name="data" type="Object"/>
<aura:attribute name="columns" type="List"/>
<aura:attribute name="comLat" type="string"/>
<aura:attribute name="comLong" type="string"/>
<lightning:notificationsLibrary aura:id="notifLib"/>
<!-- <aura:attribute name="RecId" type="String"/>-->
<aura:handler name="init" action="{!c.doInit}" value="{!this}"/>
<aura:handler name="init" action="{!c.do}" value="{!this}"/>
<!-- <lightning:button variant="brand" label="Refresh" title="Refresh" onclick="{! c.refresh }" />-->
<div class="slds-grid slds-gutters slds-wrap">
<div class="slds-col slds-small-size--1-of-1 slds-medium-size--1-of-1 slds-large-size--1-of-1 slds-show_large">
<lightning:card title=" Account Table" class="slds-card" aura:id ="changeTable">
<lightning:datatable
aura:id="tDataTable"
columns="{! v.columns }"
data="{! v.data }"
keyField="Id"
showRowNumberColumn="true"
hideCheckboxColumn="true"
onrowaction="{! c.handleRowAction }" />
</lightning:card>
</div>
</div>
</aura:component>
JS Controller:
({
doInit : function(component, event, helper) {
component.set('v.columns', [
{label: 'Name', fieldName: 'Name', type: 'text'},
{label:'Distance(KM)',type:'button',initialWidth: 135,typeAttributes:
{label: 'Distance',name: 'Distance', title: 'Click to check distance'}
},
{label:'Redirect to Map',type:'button',initialWidth: 200,typeAttributes:
{label: 'Goto Map',name: 'reDirect', title: 'Click to Visit'}
},
]);
navigator.geolocation.getCurrentPosition(function(position){
component.set("v.comLat",position.coords.latitude);
component.set("v.comLong",position.coords.longitude);
console.log('l1--'+position.coords.latitude+'---l2---'+position.coords.longitude);
});
console.log('l1--'+component.get("v.comLat")+'---l2---'+component.get("v.comLong"));
helper.fetchAccount(component, event, helper);
},
do: function (component, event, helper) {
component.set("v.LoadSpinner",true);
navigator.geolocation.watchPosition(function(position){
component.set("v.comLat",position.coords.latitude);
component.set("v.comLong",position.coords.longitude);
var l1 = component.get("v.comLat");
var l2 = component.get("v.comLong");
console.log('l1--'+l1+'---l2---'+l2);
component.set("v.LoadSpinner",false);
});
},
handleRowAction: function (component, event, helper) {
var action = event.getParam('action');
var row = event.getParam('row');
switch (action.name) {
case 'Distance':
helper.showDistance(component,row);
break;
case 'reDirect':
helper.gotoMap(component,row);
break;
}
}
})
Helper
({
fetchAccount : function(component, event, helper) {
var action = component.get("c.fetchAccounts");
action.setCallback(this,function(response) {
var state = response.getState();
if (state === "SUCCESS") {
component.set("v.data", response.getReturnValue());
// component.set("v.LoadSpinner",false);
}
});
$A.enqueueAction(action);
},
gotoMap : function(component,row){
var l1 = component.get("v.comLat");
var l2 = component.get("v.comLong");
window.open("https://www.google.com/maps/dir/?api=1&origin="+l1+","+l2+"&destination="+row.Geolocation__Latitude__s+", "+row.Geolocation__Longitude__s+"&travelmode=driving");
},
showDistance: function(component,row){
var l1 = component.get("v.comLat");
var l2 = component.get("v.comLong");
var radlat1 = Math.PI * l1/180;
var radlat2 = Math.PI * row.Geolocation__Latitude__s/180;
var theta = l2-row.Geolocation__Longitude__s;
var radtheta = Math.PI * theta/180;
var dist = Math.sin(radlat1) * Math.sin(radlat2) + Math.cos(radlat1) * Math.cos(radlat2) * Math.cos(radtheta);
if (dist > 1) {
dist = 1;
}
dist = Math.acos(dist);
dist = dist * 180/Math.PI;
dist = dist * 60 * 1.1515;
dist = dist * 1.609344;
console.log("Distance----="+dist);
component.find('notifLib').showNotice({
"variant": "success",
"header": "Account Distance",
"message": "Account is "+ dist + " KM away"
})
}
})
Apex Controller
public class GoogleMapNavigationController {
@AuraEnabled
public static List<Account> fetchAccounts(){
return [SELECT
Id, Name,Geolocation__Latitude__s,Geolocation__Longitude__s
FROM Account];
}
}
Replace geolocation custom field in Apex Controller with your custom field.
[/vc_column_text][/vc_column][/vc_row]
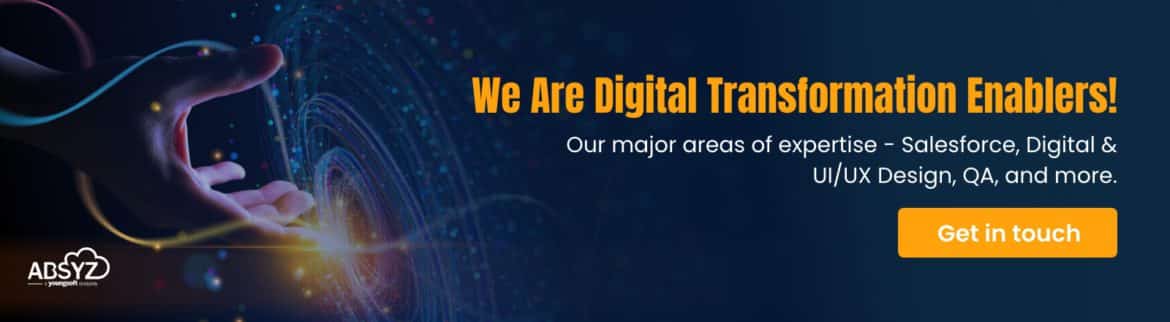