In today’s dynamic digital landscape, mastering the skill of how to generate a ZIP file using JSZIP in LWC within Salesforce is crucial for providing users with a seamless and efficient means of downloading well-organized data. Learn the step-by-step process of using JSZip in Lightning Web Components (LWC) to conveniently bundle and distribute multiple files. This not only optimizes download size but also preserves the file structure, proving invaluable, especially when dealing with large or numerous files. You can also enhance your bandwidth efficiency and accelerate download speeds.
In our prior blog post, we explored the creation of a PDF document in Lightning Web Components (LWC) using JSPDF. Now, in this installment, let’s delve into the process of downloading multiple files as a zip file. We’ll leverage the same LWC component introduced in the previous blog, showcasing seamless continuity in our exploration of versatile file handling within the LWC framework. Let’s start
STEP 1:
To initiate the file generation process as a ZIP, the essential JSZIP and JSSAVER libraries are required. Download the files in JS format from the provided links below and add them as static resources in your Salesforce org.
JSZip Link: https://github.com/Stuk/jszip/blob/main/dist/jszip.min.js
JSSaver Link: https://cdnjs.cloudflare.com/ajax/libs/FileSaver.js/2.0.0/FileSaver.min.js
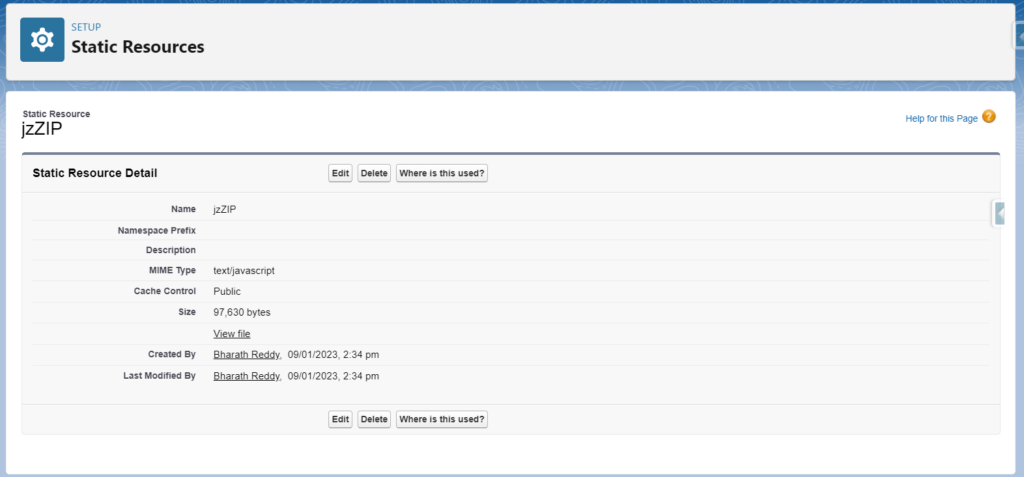
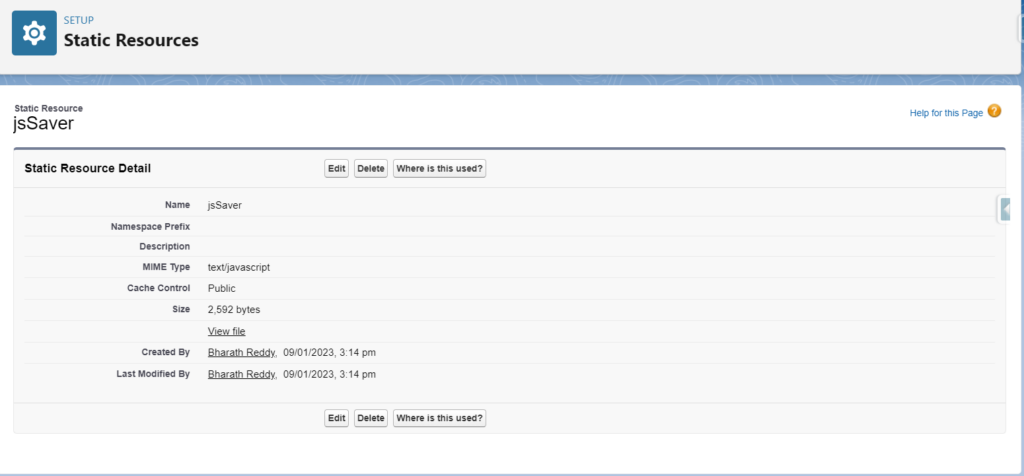
STEP 2:
In our earlier blog, we successfully created a PDF file. Now, in the current discussion, we will extend our capabilities to generate a zip file and include additional files within it. Let’s revisit our previous Lightning Web Component (LWC) and enhance its functionality to accommodate the creation of a zip file with added files.
STEP 3: (Explanation)
3.1:
Import the JSZIP and JSSAVER files what we added in static resource as below in LWC JS file
import jsZip from '@salesforce/resourceUrl/jzZIP';
import jsSaver from '@salesforce/resourceUrl/jsSaver';
3.2:
To load external JavaScript libraries (jsZip and jsSaver) dynamically into the component use the loadScript function as below
renderedCallback(){
loadScript(this, jsZip ).then(() => {});
loadScript(this, jsSaver ).then(() => {});
if (this.jsPdfInitialized) {
return;
}
this.jsPdfInitialized = true;
}
3.3 :
Create a method and write an instance of JSZIP and then add files to it.
generateZIP(){
var zip = new JSZip();
zip.file("contactData.pdf", “doc.output('blob')”); // Added previous blog generated pdf
zip.file("Hello.txt", "Hello World\n");
zip.file("Hello.xls", "Hello World\n");
zip.generateAsync({ type: "blob", })
.then(function (content) {
saveAs(content, "contact data.zip");
3.4:
To make the code more structured and easier, call the generateZIP() method in another method.
generateData(){
this.generateZip();
}
3.5:
We know that we already placed our button in the record page . We will download pdf along with new added docs
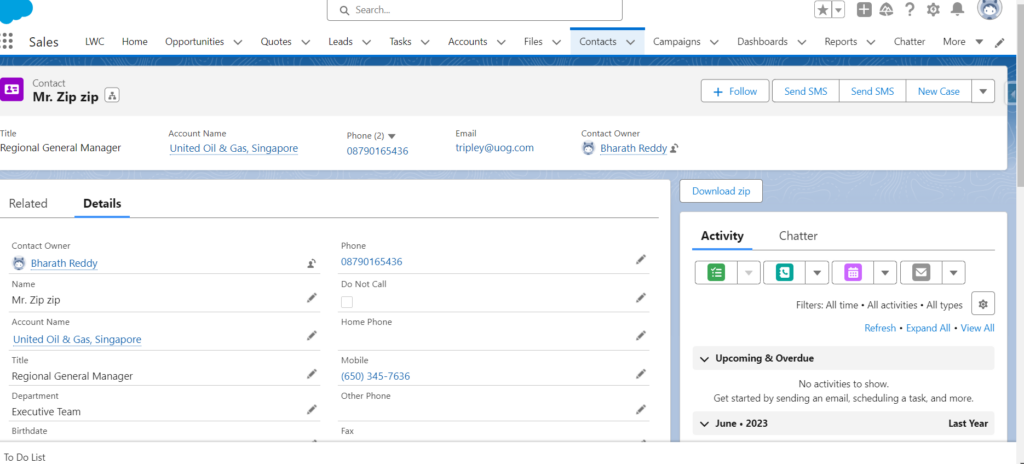
3.6:
Click on the “download zip” button to see the output.
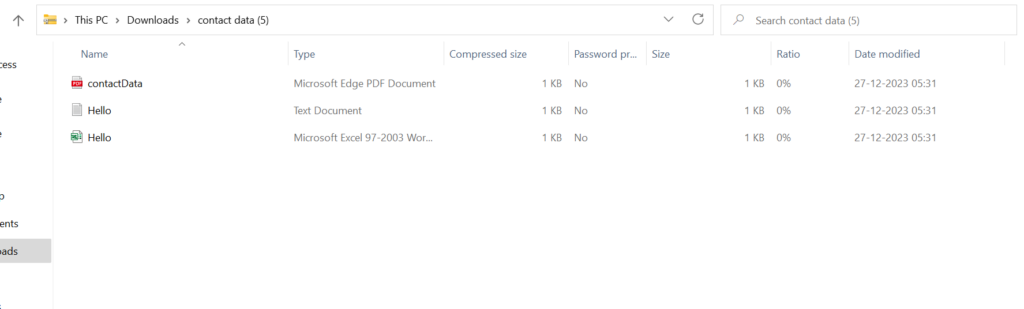
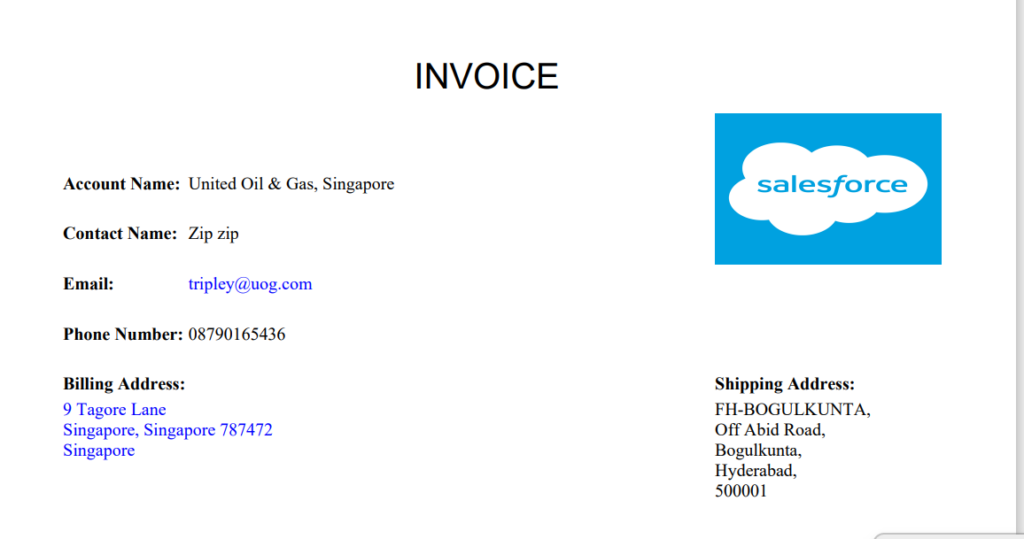
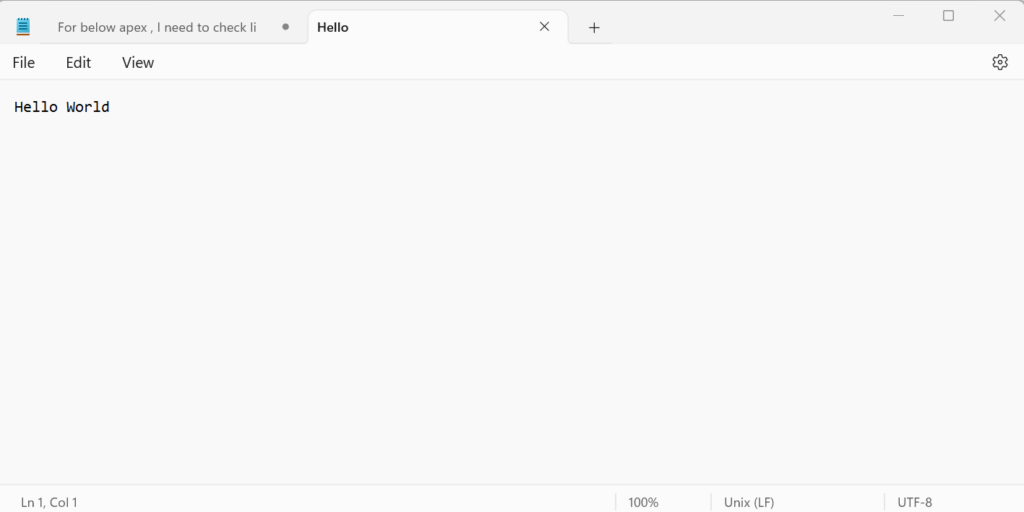
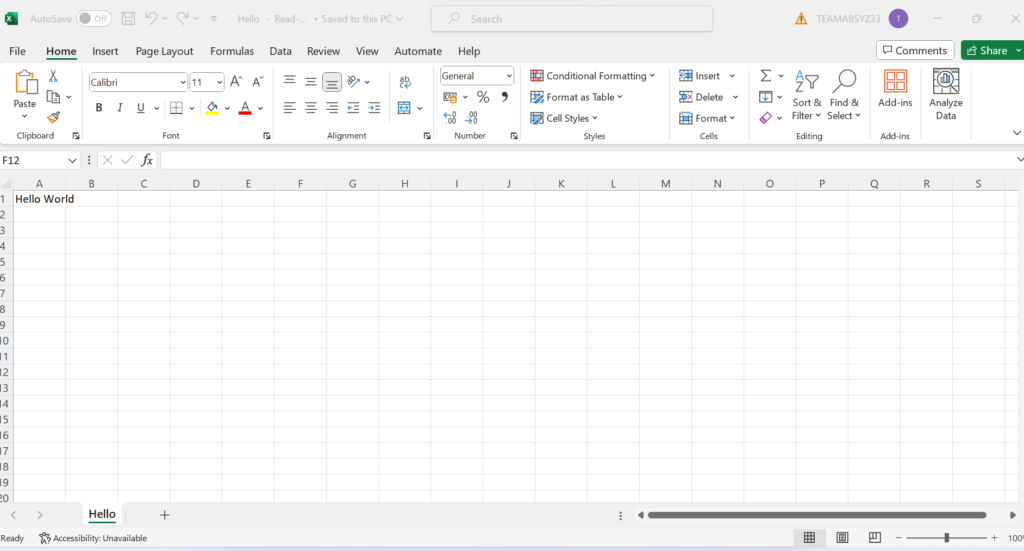
STEP 4: Revised Code- Reusing the same old LWC.
HTML Code:
JS Code:
import { LightningElement,api,wire,track } from 'lwc';
import { getRecord, getFieldValue } from "lightning/uiRecordApi";
const FIELDS = [
'Contact.Id','Contact.Name', 'Contact.Account.Name', 'Contact.Phone',
'Contact.MailingStreet', 'Contact.OtherStreet', ' Contact.Email'
];
import jsPDF from '@salesforce/resourceUrl/jsPDF';
import jsZip from '@salesforce/resourceUrl/jzZIP';
import jsSaver from '@salesforce/resourceUrl/jsSaver';
import { loadScript } from 'lightning/platformResourceLoader';
export default class GeneratePDF extends LightningElement {
@track name;
@track accountName;
@track email;
@track billTo;
@track shipTo;
@track phone;
@api recordId;
@wire(getRecord, {
recordId: "$recordId",
fields:FIELDS
})
contactData({data,error}){
if(data){
console.log('data'+JSON.stringify(data))
this.name=getFieldValue(data,'Contact.Name');
this.accountName=getFieldValue(data,'Contact.Account.Name')
this.email=getFieldValue(data,'Contact.Phone')
this.billTo=getFieldValue(data,'Contact.MailingStreet')
this.shipTo=getFieldValue(data,'Contact.OtherStreet')
this.phone=getFieldValue(data,'Contact.Email')
}
else if(error){
console.log(error)
}
}
jsPdfInitialized=false;
renderedCallback(){
loadScript(this, jsPDF ).then(() => {});
loadScript(this, jsZip ).then(() => {});
loadScript(this, jsSaver ).then(() => {});
if (this.jsPdfInitialized) {
return;
}
this.jsPdfInitialized = true;
}
generateZIP(){
const { jsPDF } = window.jspdf;
const doc = new jsPDF();
Var imgData=’Base 64 image URL’
doc.addImage(imgData, 'PNG', 150, 25, 45, 30);
doc.setFontSize(20);
doc.setFont('helvetica')
doc.text("INVOICE", 90, 20,)
doc.setFontSize(10)
doc.setFont('arial black')
doc.text("Account Name:", 20, 40)
doc.text("Contact Name:", 20, 50)
doc.text("Phone Number:", 20, 60)
doc.text("Email:", 20, 70)
doc.text("Billing Address:", 20, 80)
doc.text("Shipping Address:", 150, 80)
doc.setFontSize(10)
doc.setFont('times')
doc.text(this.accountName, 45, 40)
doc.text(this.phone, 45, 70)
doc.text(this.name, 45, 50)
doc.text(this.billTo, 20, 85)
doc.text(this.shipTo, 150, 85)
doc.text(this.email, 45, 60)
doc.output()
var zip = new JSZip();
zip.file("contactData.pdf", doc.output('blob')); // If you didn’t added image means remove ‘blob’
zip.file("Hello.txt", "Hello World\n");
zip.file("Hello.xls", "Hello World\n");
zip.generateAsync({ type: "blob", })
.then(function (content) {
saveAs(content, "contact data.zip");
}
generateData(){
this.generateZIP();
}
}
Meta-xml code:
55.0
true
lightning__RecordPage
📄 doc.text(“INVOICE”, 90, 20,) means doc.text(“text”,length from page left, length from page top)
📄 If you want to add image, convert your image into base64 Url from
🔗 https://elmah.io/tools/base64-image-encoder/
📄 doc.addImage(imgData, ‘PNG’, 150, 25, 45, 30) means doc.addImage(imageVariable,type of image,length from page left, length from page top,length of image,width of image)
Conclusion
In this blog post, we delve into the practical implementation of how to generate a ZIP file using JSZIP in LWC. Demonstrating the synergy between jsPDF and JSZip, we showcase a seamless user experience for downloading contact-related documents. This example not only highlights the power of Lightning Web Components but also serves as a step-by-step guide on how to enhance Salesforce applications by efficiently generating and downloading Zip archives.