Generating PDFs is a common requirement in business applications, and it is often useful to allow users to generate PDF using JSPDF in LWC from their data with just one click. We generate pdf for many cases like downloading invoices, downloading reports and if you want to export data from certain objects etc. we can use a VisualForce page to download a PDF document.
In this article, we will take a closer look at how to generate a PDF using (Lightning Web Components) LWC and the JSPDF library. Generating PDFs is now possible with minimal efforts using JSPDF. The code can be easily adapted to generate PDFs of other records or data.
STEP 1: Go to the link below, download the file and upload it in Salesforce static resource with name “jspdf”
🔗 https://github.com/parallax/jsPDF/blob/master/dist/jspdf.umd.min.js
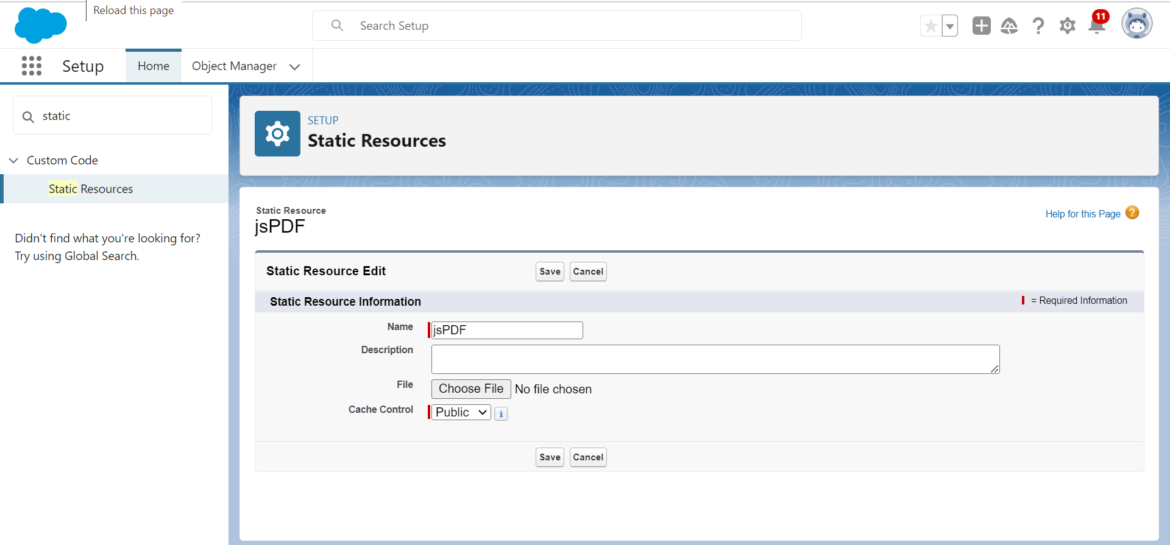
STEP 2: Create a new LWC component.
STEP 3: Explanation
3.1 Import the file that is downloaded from js library(step -1) and Import loadscript file which is available for salesforce.
import jsPDF from '@salesforce/resourceUrl/jsPDF';
import { loadScript } from 'lightning/platformResourceLoader';
3.2 Use renderedCallBack to call loadScript
jsPdfInitialized=false;
renderedCallback(){
console.log(this.contact.data);
loadScript(this, jsPDF ).then(() => {});
if (this.jsPdfInitialized) {
return;
}
this.jsPdfInitialized = true;
}
3.3 Now create a method and write a instance of jspdf to generate pdf
generatePdf(){
const { jsPDF } = window.jspdf;
const doc = new jsPDF();
3.4 If you want to get data from record page import as below
import { getRecord, getFieldValue } from "lightning/uiRecordApi";
const FIELDS = [
'Contact.Id','Contact.Name', 'Contact.Account.Name', 'Contact.Phone',
'Contact.MailingStreet', 'Contact.OtherStreet', ' Contact.Email'
];
3.5 Get the data by using @wire method
@track name;
@track accountName;
@track email;
@track billTo;
@track shipTo;
@track phone;
@api recordId;
@wire(getRecord, {
recordId: "$recordId",
fields:FIELDS
})
contactData({data,error}){
if(data){
console.log('data'+JSON.stringify(data))
this.name=getFieldValue(data,'Contact.Name');
this.accountName=getFieldValue(data,'Contact.Account.Name')
this.email=getFieldValue(data,'Contact.Phone')
this.billTo=getFieldValue(data,'Contact.MailingStreet')
this.shipTo=getFieldValue(data,'Contact.OtherStreet')
this.phone=getFieldValue(data,'Contact.Email')
}
else if(error){
console.log(error)
}
}
3.6 Add the data from record page and the static data you want to pdf as follows
doc.text(“Billing Address:”, 20, 80)
doc.text(“Shipping Address:”, 150, 80)
doc.setFontSize(10)
doc.setFont(‘times’)
doc.text(this.accountName, 45, 40)
doc.text(this.phone, 45, 60)
doc.text(this.name, 45, 50)
doc.text(this.billTo, 20, 85)
doc.text(this.shipTo, 150, 85)
doc.text(this.email, 45, 70)
3.7 Save the pdf as
doc.save(“CustomerInvoice.pdf”)
3.8 Take another method to call above method
generateData(){
this.generatePdf();
}
3.9 In html create a button, and call this method
<template>
<lightning-button label="Download PDF" onclick={generateData}></lightning-button>
</template>
3.10 Add this button on the record page.
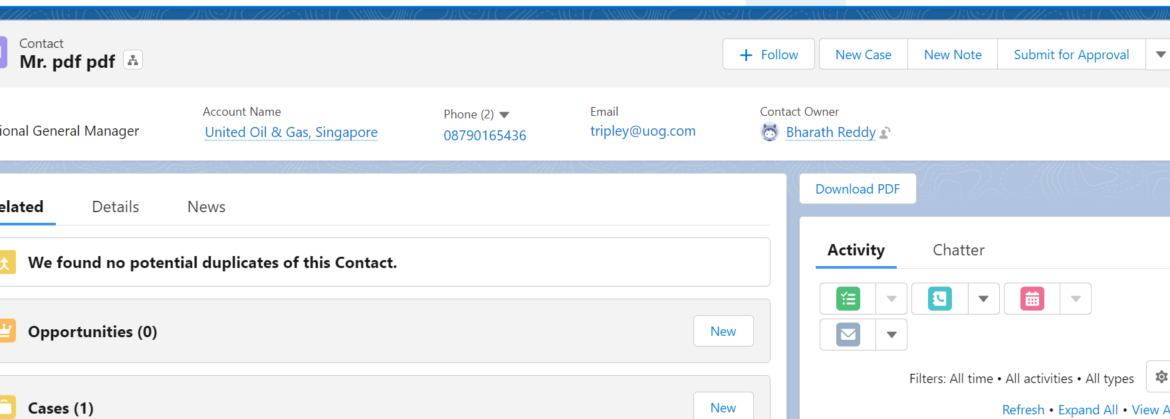
STEP 4: Click on the download button to see output
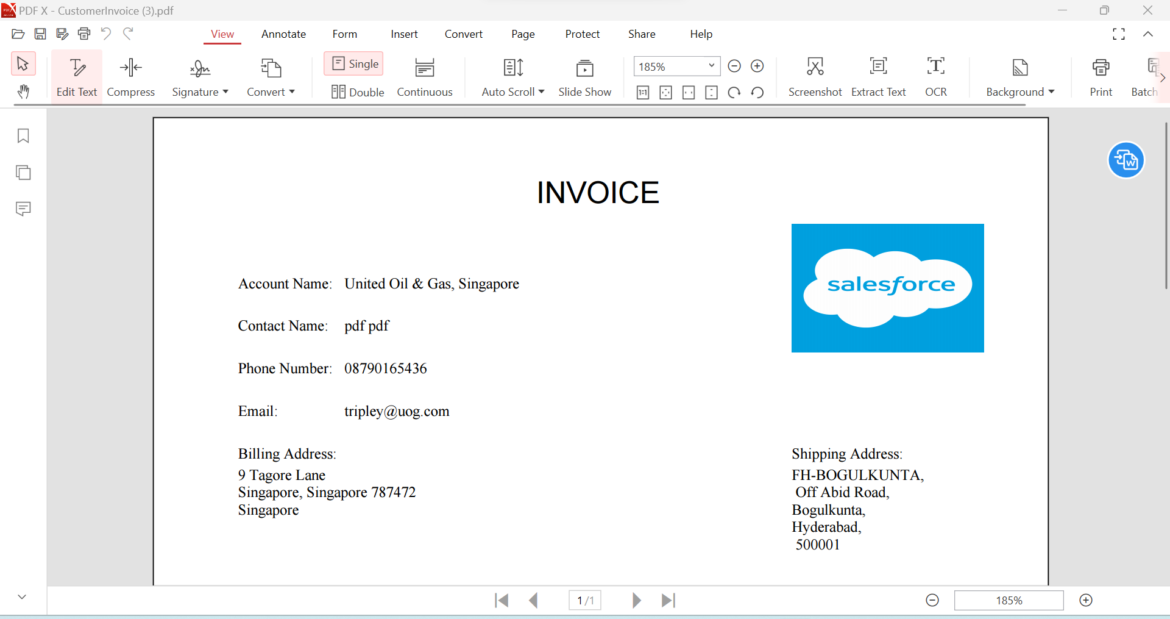
STEP 5: TOTAL CODE
HTML Code:
<template>
<lightning-button label="Download PDF" onclick={generateData}></lightning-button>
</template>
JS Code:
import { LightningElement,api,wire,track } from ‘lwc’;
import { getRecord, getFieldValue } from “lightning/uiRecordApi”;
const FIELDS = [
‘Contact.Id’,’Contact.Name’, ‘Contact.Account.Name’, ‘Contact.Phone’,
‘Contact.MailingStreet’, ‘Contact.OtherStreet’, ‘ Contact.Email’
];
import jsPDF from ‘@salesforce/resourceUrl/jsPDF’;
import { loadScript } from ‘lightning/platformResourceLoader’;
export default class GeneratePDF extends LightningElement {
@track name;
@track accountName;
@track email;
@track billTo;
@track shipTo;
@track phone;
@api recordId;
@wire(getRecord, {
recordId: “$recordId”,
fields:FIELDS
})
contactData({data,error}){
if(data){
console.log(‘data’+JSON.stringify(data))
this.name=getFieldValue(data,’Contact.Name’);
this.accountName=getFieldValue(data,’Contact.Account.Name’)
this.email=getFieldValue(data,’Contact.Phone’)
this.billTo=getFieldValue(data,’Contact.MailingStreet’)
this.shipTo=getFieldValue(data,’Contact.OtherStreet’)
this.phone=getFieldValue(data,’Contact.Email’)
}
else if(error){
console.log(error)
}
}
jsPdfInitialized=false;
renderedCallback(){
console.log(this.contact.data);
loadScript(this, jsPDF ).then(() => {});
if (this.jsPdfInitialized) {
return;
}
this.jsPdfInitialized = true;
}
generatePdf(){
const { jsPDF } = window.jspdf;
const doc = new jsPDF();
📄 doc.text(“INVOICE”, 90, 20,) means doc.text(“text”,length from page left, length from page top)
doc.addImage
📄 If you want to add image, convert your image into base64 Url from
🔗 https://elmah.io/tools/base64-image-encoder/
📄(imgData, ‘PNG’, 150, 25, 45, 30) means doc.addImage(imageVariable,type of image,length from page left, length from page top,length of image,width of image)
Var imgData=’Base 64 image URL’
doc.addImage(imgData, ‘PNG’, 150, 25, 45, 30);
doc.setFontSize(20);
doc.setFont(‘helvetica’)
doc.text(“INVOICE”, 90, 20,)
doc.setFontSize(10)
doc.setFont(‘arial black’)
doc.text(“Account Name:”, 20, 40)
doc.text(“Contact Name:”, 20, 50)
doc.text(“Phone Number:”, 20, 60)
doc.text(“Email:”, 20, 70)
doc.text(“Billing Address:”, 20, 80)
doc.text(“Shipping Address:”, 150, 80)
doc.setFontSize(10)
doc.setFont(‘times’)
doc.text(this.accountName, 45, 40)
doc.text(this.phone, 45, 60)
doc.text(this.name, 45, 50)
doc.text(this.billTo, 20, 85)
doc.text(this.shipTo, 150, 85)
doc.text(this.email, 45, 70)
doc.save(“CustomerInvoice.pdf”)
}
generateData(){
this.generatePdf();
}
}
Meta-xml code:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>55.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
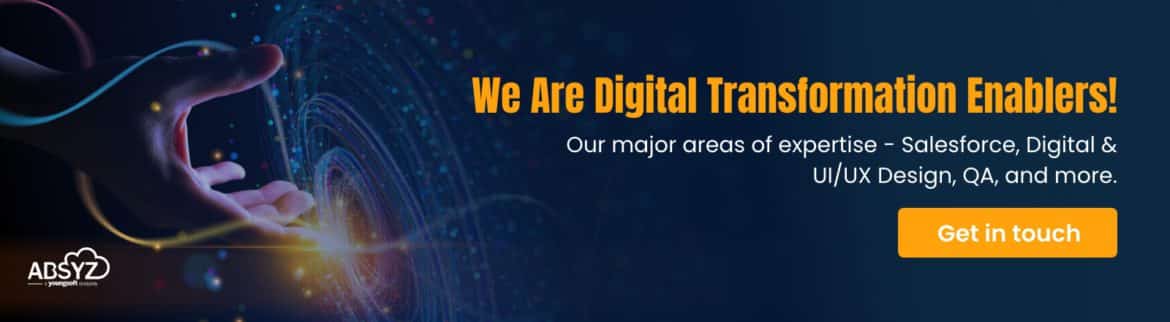