Exceptions are bad unless we know what actually caused them. My personal experience with exceptions has always been quite bad. It becomes worse if you are working with Lightning Components.
Errors happen. Sometimes they’re expected, such as invalid input from a user, etc. and sometimes they just appear out of the blue, leaving you clueless.
Now working with Lightning components, you can come across two types of exceptions:
- Client side
- Server side
When your server-side controller code experiences an error, two things can happen. You can catch it there and handle it in Apex. Otherwise, the error is passed back in the controller’s response.
If you handle the error Apex, you again have two ways you can go. You can process the error, perhaps recovering from it, and return a normal response to the client. Or, you can create and throw an AuraHandledException.
Exceptions, when not handled properly at the server side, will end up displaying errors like below.
Now, to overcome such situations, we can make use of AuraHandledException. Its use gives us a chance to handle the exception more gracefully in our client code. System exceptions do not contain important details for security purposes, and always result in the dreaded “An internal server error has occurred…” message as shown above. I don’t think anyone of us likes that.
But if we make use of AuraHandledException, we can handle the exception occurring at the server side as well as return a more customised message to be displayed at the client side.
Now let’s see how to use AuraHandledExceptions in our code with the help of a basic example.
Consider a simple scenario of displaying Account records in a table. Consider the below code:
Component:
Controller :
[code lang=”javascript”]
({
showRecs: function(component, evt) {
var action = component.get(“c.returnRecords”);
console.log(“hi”);
action.setCallback(this, function(a) {
var state = a.getState();
if (state == ‘SUCCESS’) {
component.set(“v.accRecs”, a.getReturnValue());
}
});
$A.enqueueAction(action);
}
})
[/code]
Handler :
Now, in the above scenario, the code will return a runtime exception in the server side, as the field Email is not present in the standard Account object. But at the client side, it won’t display any error or message as such. In such scenarios, it is hard to find out the reasons causing the exceptions unless the debug logs are enabled.
Thus we use AuraHandledExceptions so that the client gets a better idea and as well as can handle the exceptions occurring at the server side better.
The above code can be modified as below :
Controller :
[code lang=”javascript”]
({
showRecs: function(component, evt) {
var action = component.get(“c.returnRecords”);
console.log(“hi”);
action.setCallback(this, function(a) {
var state = a.getState();
if (state == ‘SUCCESS’) {
component.set(“v.accRecs”, a.getReturnValue());
} else {
var errorMsg = action.getError()[0].message;
console.log(errorMsg);
var toastEvent = $A.get(“e.force:showToast”);
toastEvent.setParams({
title: ‘Error’,
type: ‘error’,
message: errorMsg
});
toastEvent.fire();
}
});
$A.enqueueAction(action);
}
})
[/code]
Handler :
Output:
In the above case, the AuraHandledException is sent back to the client with my custom message, and I have displayed the exception using a toast. You can display it using any other method such as Modals, etc.
This was one such case. You can also display custom exceptions using the throw statement. For eg.
Thus we can now customise the messages the client see in case of an exception.For more such examples, click here.
Happy coding!!
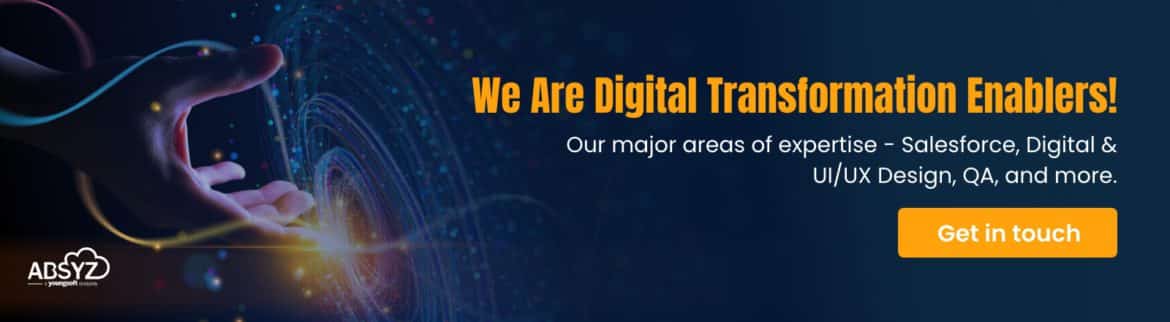