It is often useful for a user to keep track updates on the case feed this will helps to resolve the cases more efficiently. Salesforce provides “Follow” feature, Using this feature we can track updates to a record, including field changes, posts, tasks, and comments on records.
If the user follows a record, he can see in his feed depend on which of the fields your administrator configured for feed tracking and he will get updates according to his email settings.
Follow the below steps to enable Chatter Email Settings or Email Notifications :
- Go to Name and click on SetUp.
- Click on My Settings.
- Search Chatter in the Quick Find box.
- Click on Email Notifications and Check/Uncheck checkboxes.
Limitations with Standard Salesforce Follow Feature:
- we can’t see Updates to encrypted custom fields in feeds.
- we can follow a maximum combined total of 500 people, topics, and records.
We built a custom “Follow” Functionality which will work like Standard Salesforce Follow functionality but this can be used in any Lightning Page and it is generic component.
Components List:
- DisplayCaseRecords
- FollowOrUnFollowRecord(Generic Component)
Apex Controller:
- FollowUnFollowRecordController
After clicking on the Follow button
After mouse over on Following button
Take a look at the below components
DisplayCaseRecords.cmp
This component is used to display case records and Follow / UnFollow records.
[sourcecode language=”java”]</pre>
<aura:component controller=”FollowUnFollowRecordController” implements=”force:appHostable, flexipage:availableForAllPageTypes, forceCommunity:availableForAllPageTypes, force:lightningQuickAction” access=”global” >
<!– Internal Attributes –>
<aura:attribute name=”caseWrapList” type=”Object[]” />
<!– Handlers –>
<aura:handler name=”init” value=”this” action=”{!c.doInit}”/>
<table class=”slds-table slds-table_bordered slds-table_cell-buffer”>
<thead>
<tr class=”slds-text-title_caps”>
<th scope=”col”>
<div class=”slds-truncate” title=”Subject”> Subject</div></th>
<th scope=”col”>
<div class=”slds-truncate” title=”Status”> Status</div></th>
<th scope=”col”>
<div class=”slds-truncate” title=”Priority” > Priority</div></th>
</tr>
</thead>
<aura:iteration items=”{!v.caseWrapList}” var=”caseWrap” >
<tbody>
<tr>
<td data-label=”Subject” >
<div class=”slds-truncate” title=”Subject” > {!caseWrap.caseRec.Subject}</div></td>
<td data-label=”Status”>
<div class=”slds-truncate” title=”Status”> {!caseWrap.caseRec.Status}</div></td>
<td data-label=”Priority”>
<div class=”slds-truncate” title=”Priority”> {!caseWrap.caseRec.Priority}</div></td>
<td>
<lightning:buttonGroup >
<c:FollowOrUnFollowRecord recordId=”{!caseWrap.caseRec.Id}” isFollowed=”{!caseWrap.isFollowed}”/>
<lightning:button value=”{!caseWrap.caseRec.Id}” variant=”neutral” label=”More Info” iconPosition=”left” onclick=”{!c.navigateTOCaseRecord}” />
</lightning:buttonGroup></td>
</tr>
</tbody>
</aura:iteration></table>
</aura:component>
<pre>[/sourcecode]
DisplayCaseRecordsController.js
[sourcecode language=”java”]
({
doInit : function(component, event, helper) {
helper.getCaseRecords(component);
},
navigateTOCaseRecord : function(component, event, helper) {
var recordId=event.getSource().get(“v.value”);
window.open(‘/’ + recordId);&amp;nbsp;&amp;nbsp;&amp;nbsp; &amp;nbsp;
}
})
[/sourcecode]
DisplayCaseRecordsHelper.js
[sourcecode language=”java”]({
getCaseRecords : function(component) {
var action=component.get(“c.getCaseRecords”);
action.setCallback(this, function(response) {
var state = response.getState();
if (state === “SUCCESS”){
component.set(“v.caseWrapList”,response.getReturnValue());
}
else if(state === “ERROR”){
alert(‘Problem with connection. Please try again.’);
}
});
$A.enqueueAction(action);
}
})
[/sourcecode]
FollowOrUnFollowRecord.cmp
This is the Generic component, In which I am using lightning:buttonStateful component represents a button which will toggle between states. By default “Follow” will display, “Following” will display when we click on the button. We can use icons along with text label but It supports only Utility category icons.
[sourcecode language=”java”]<aura:component controller=”FollowUnFollowRecordController” access=”global” >
<!– External Attributes –>
<aura:attribute name=”recordId” type=”string” description=”the record Id of the any object” />
<aura:attribute name=”isFollowed” type=”Boolean” default=”false” description=”check if the record is already followed by current user”/>
<!– Component Core –>
<lightning:buttonStateful labelWhenOff=”Follow” labelWhenOn=”Following”
labelWhenHover=”Unfollow”
iconNameWhenOff=”utility:add”
iconNameWhenOn=”utility:check”
iconNameWhenHover=”utility:close”
state=”{!v.isFollowed}”
onclick=”{!c.follow }”
/>
</aura:component>[/sourcecode]
FollowOrUnFollowRecordController.js
[sourcecode language=”java”]({
follow : function(component, event, helper) {
helper.followRecord(component);
}
})
[/sourcecode]
FollowOrUnFollowRecordHelper.js
[sourcecode language=”java”]({
// followRecord Method used to Follow/Unfollow a record
followRecord : function(component) {
var recordId = component.get(“v.recordId”);
var isFollowed = component.get(“v.isFollowed”);
var action=component.get(“c.followRecord”);
action.setParams({
“recordId” : component.get(“v.recordId”)
“isFollowed” : component.get(“v.isFollowed”)
});
action.setCallback(this, function(response) {
var state = response.getState();
if (state === “SUCCESS”)
{
component.set(“v.isFollowed”,response.getReturnValue())
}
else if(state === “ERROR”)
{
alert(‘Problem with connection. Please try again.’);
}
});
$A.enqueueAction(action);
}
})
&lt;span&gt;[/sourcecode]
FollowUnFollowRecordController.apexc
[sourcecode language=”java”]
public class FollowUnFollowRecordController {
// To store case Ids.
public static set<Id> caseIds {get;set;}
// This method is used to check the record is already followed by current user or not.
public static Set<Id> getMyFollowedAccntId() {
if (caseIds == null){
List<EntitySubscription> lstEntitySubscription = new List<EntitySubscription>();
caseIds = new set<ID>();
lstEntitySubscription = [SELECT ParentId FROM EntitySubscription WHERE SubscriberId =: UserInfo.getUserId() AND Parent.Type =’Case’ ORDER By CreatedDate DESC LIMIT 1000];
for (EntitySubscription entitysubscription :lstEntitySubscription){
caseIds.add(entitysubscription.ParentId);
} } return caseIds;
} // This method is used to return the case Records.
@AuraEnabled
public static List<caseWrapper> getCaseRecords() {
List<caseWrapper> caseWrapList = new List<caseWrapper>();
for(case ca: [Select id,subject,status,priority,caseNumber from case limit 10 ]){
caseWrapList.add(new caseWrapper(ca, getMyFollowedAccntId().contains(ca.id) ));
} return caseWrapList; }
//This method is used to Follow/UnFollow record.
@AuraEnabled
public static Boolean followRecord(String recordId,Boolean isFollowed) {
try{
if(!isFollowed){
EntitySubscription entitysubscription = new EntitySubscription(parentId=recordId, SubscriberId=UserInfo.getUserId());
insert entitysubscription;
}
if(isFollowed){
EntitySubscription entitysubscription = [SELECT id FROM EntitySubscription WHERE parentId =: recordId AND subscriberId =: UserInfo.getUserId()];
delete entitysubscription;
}
return !isFollowed;
}catch(Exception except){
system.debug(except);
return null;
}
return true;
}
/* * @desc Case wrapper class */
public class caseWrapper{
@AuraEnabled public Case caseRec {get;set;}
@AuraEnabled public Boolean isFollowed {get;set;}
// constructor
public caseWrapper(){}
// constructor with paramters.
public caseWrapper(Case caseRec, Boolean isFollowed){
this.caseRec = caseRec;
this.isFollowed = isFollowed;
}
}
}[/sourcecode]
Please feel free to comment.
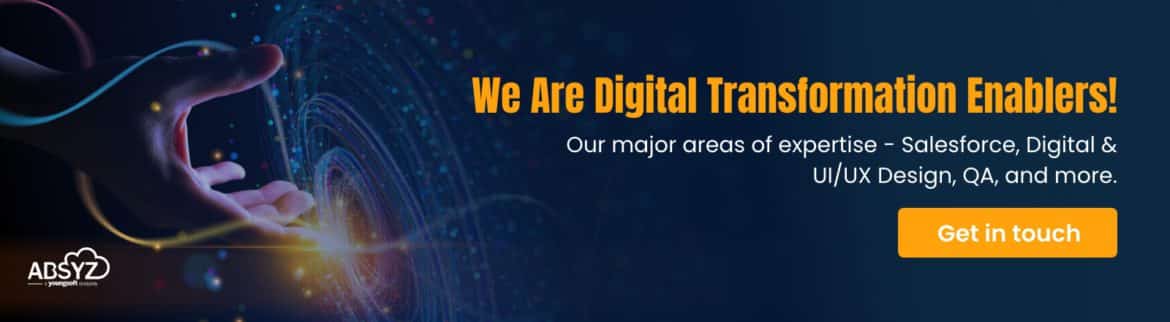