Einstein Intent
Einstein Intent helps to categorize unstructured data into labels using its Intent API for a better understanding of what user is trying to accomplish. In Salesforce, Einstein Intent API allows understanding the customer inquiries that is easy to automatically route leads, escalate service and personalize marketing. This is helpful in prioritizing customer service inquiries. For example, Einstein Language comes into Salesforce where Einstein Intent and Einstein Sentimental analysis are in use by including them in chatter. Here in Salesforce Chatter, the post where the Einstein Intent and the comments where Einstein Sentiment can be applied to the real world example of e-commerce that is discussed below.
For a better understanding of Einstein Intent, you can refer the Intent basics trailhead (https://trailhead.salesforce.com/en/modules/einstein_intent_basics). The steps to access Einstein Intent are:
- Create Dataset
- Train the Dataset
- Predict
Create Dataset:
Create a .csv file with a column of sample data and another column as labels as shown below. You can create your own dataset and use the downloadable link for training data. To know more about how to create a downloadable link you can refer our blog. The more data you give the more Einstein is getting trained to be accurate. Here an e-commerce example is used with the labels named: Billing, Password Help, Shipping Info, Order Change and Sales Opportunity.
Train the Dataset:
We give the downloadable link in the URL field and train dataset. In detail, refer the blog for training the data in a step by step process to create component where models are created to predict the text. After you follow the steps, go to setup -> apex classes and replace the code because we are calling the Intent API to train the data. You find the classes like this.
In the class, EinsteinVision_PredictionService.apex the intent is passed to the API language added to the URL. Here the change the endpoint URL as
[sourcecode language=”java”]
private static String BASE_URL = ‘https://api.einstein.ai/v2’;
private String DATASETS = BASE_URL + ‘/language/datasets’;
private String LABELS = ‘/labels’;
private String EXAMPLES = ‘/examples’;
private String TRAIN = BASE_URL + ‘/language/train’;
private String MODELS = ‘/language/models’;
private String PREDICT = BASE_URL + ‘/language/intent’;
private String API_USAGE = BASE_URL + ‘/apiusage’;
private static String OAUTH2 = BASE_URL + ‘/oauth2/token’;
public String data;
[/sourcecode]
Change the URL value as ‘text-intent’ in all the methods
[sourcecode language=”java”]
EinsteinVision_HttpBodyPartDatasetUrl parts = new EinsteinVision_HttpBodyPartDatasetUrl(url,’text-intent’);
[/sourcecode]
And the parameter to pass is only a string so that change the argument name where ever necessary to pass the model id and data.
[sourcecode language=”java”]
public EinsteinVision_PredictionResult METHOD (String modelId, String data) {
EinsteinVision_HttpBodyPartPrediction parts = new EinsteinVision_HttpBodyPartPrediction(modelId, data);
[/sourcecode]
In the EinsteinVision_HttpBodyPartPrediction.apex class change the parameter to pass.
[sourcecode language=”java”]
public EinsteinVision_HttpBodyPartPrediction(String modelId, String data) {
this.modelId = modelId;
this.data = data;
}
[/sourcecode]
Now the apex class is ready to use for Einstein Intent. The sample data can be downloaded from this link and the same can be specified to train the data (http://einstein.ai/text/case_routing_intent.csv).
Predict:
Here when I give a text, Einstein Intent will find the probability which type of department it belongs to and categorizes the data. Pass the CSV file name that you have saved it or refer the dataset name after training that is shown in the above image.
[sourcecode language=”java”]
@AuraEnabled
public static String getPrediction(String Comments) {
EinsteinVision_PredictionService service = new EinsteinVision_PredictionService();
EinsteinVision_Dataset[] datasets = service.getDatasets();
String Prob;
for (EinsteinVision_Dataset dataset : datasets) {
if (dataset.Name.equals(‘case_routing_intent.csv’)) {
EinsteinVision_Model[] models = service.getModels(dataset);
EinsteinVision_Model model = models.get(0);
EinsteinVision_PredictionResult result = service.predictBlob(model.modelId, Comments);
EinsteinVision_Probability probability = result.probabilities.get(0);
Prob = result.probabilities.get(0).label;
}
}
return Prob;
}
[/sourcecode]
The component to get the data from the user and display the probability.
[sourcecode language=”html”]
<aura:component controller=”EinsteinVision_Admin” implements=”force:appHostable, flexipage:availableForAllPageTypes, flexipage:availableForRecordHome, force:hasRecordId, forceCommunity:availableForAllPageTypes, force:lightningQuickAction” access=”global”>
<aura:attribute name=”CsvURL” type=”String”/>
<aura:attribute name=”Probablity” type=”Object”/>
<center>
<lightning:card >
<aura:set attribute=”title”>
<p style=”font-family:serif;font-size: 40px;”>User Feedback</p>
</aura:set>
<div style=”width:40%;”>
<ui:inputText aura:id=”select” placeholder=”Enter your comments”/><br/>
<br/>
<lightning:button onclick=”{!c.extractfile}”>search</lightning:button><br/>
<br/>
</div>
<p style=”font-family:serif;font-size: 20px;”><ui:outputTextArea value=”{!v.Probablity}”/></p>
</lightning:card>
</center>
</aura:component>
[/sourcecode]
Pass the data form user to apex controller using controller.js and return the response.
[sourcecode language=”java”]
({
extractfile : function(component, event, helper) {
var action = component.get(“c.getPrediction”);
var text = component.find(“select”).get(“v.value”);
action.setParams({
Comments: text
});
action.setCallback(this, function(response) {
component.set(“v.waiting”, false);
var state = response.getState();
if (state === ‘ERROR’) {
var errors = response.getError();
if (errors) {
if (errors[0] && errors[0].message) {
return alert(errors[0].message);
}
} else {
return console.log(“Unknown error”);
}
}
var result = response.getReturnValue();
component.set(“v.Probablity”,result);
});
component.set(“v.waiting”, true);
$A.enqueueAction(action);
}
})
[/sourcecode]
The intent of the text that we get is the output shown below.
Salesforce Chatter
The real-time example where we can make use of Einstein Language on Salesforce is the Chatter. Here we are posting a message to calculate the Intent and the comments to the post made by the users to calculate the Sentiment (Positive, Neutral and Negative). When a user posts a message on Chatter and a comment is given to it, a trigger is fired to Intent and Sentiment methods. I have a created a custom object to store the posts and comments in the custom fields. On after insert trigger, we feed the text to the apex methods to get the type and sentiment of the text.
Trigger to fire after insert on FeedComment:
[sourcecode language=”java”]
trigger Einstein_ChatterComment on FeedComment (after insert) {
String Body;
For(FeedComment comment : Trigger.New)
{
Body = comment.Id;
}
Einstein_ChatterHandler.getProbability(Body);
}
[/sourcecode]
Handler for the trigger:
[sourcecode language=”java”]
global class Einstein_ChatterHandler {
@future(callout=true)
Public static void getProbability(String body){
FeedComment fd=[select CommentBody, FeedItemId from FeedComment where id=:body];
FeedItem fi = [select Body from FeedItem where id=:fd.FeedItemId];
String intentLabel = EinsteinVision_Admin.getPrediction(fi.Body);
String sentimentLabel = EinsteinVision_Sentiment.findSentiment(fd.CommentBody);
Einstein__c ein = new Einstein__c(Chatter_Message__c=fi.body, Comment__c=fd.CommentBody, Feedback_type__c=intentLabel, Emotion__c=sentimentLabel);
insert ein;
}
}
[/sourcecode]
Finally, we create reports to view the analyzed data as shown below. The report is grouped by sentiment for each department.
Einstein Language can be implemented on social media to know the customer view that will be our upcoming blog. Please feel free to contact us for any doubts and queries.
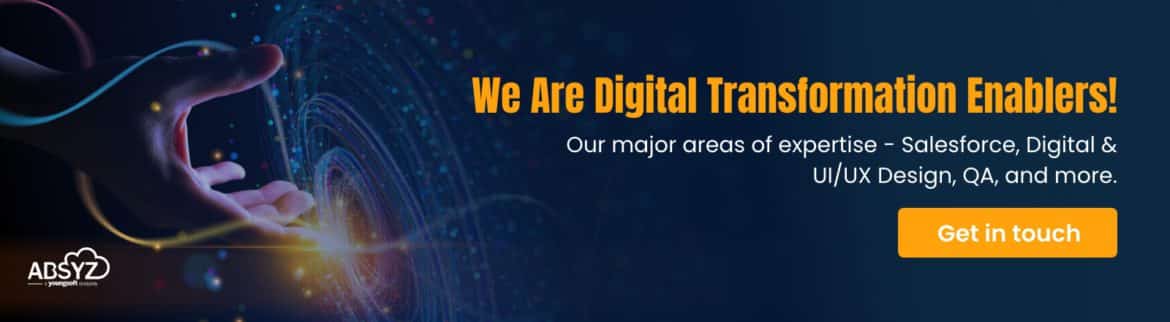