Hi All,
I was trying to build a modal dialog box in lightning component and it took me some time to figure out how to use svg tag and use it in Lightning component.
In this blog, I will try to create a Account List Lightning Component which will have a new Account create button.The new button will open a form dialog box which will contain the Account fields.On the click of Save the Account record will be created.
Attached is the screen of the Lightning App once you complete this tutorial.
Let’s first create a lightning component which shows a list of accounts
AccountListController.apxc :
[sourcecode language=”java”]
public class AccountListController {
@AuraEnabled
public static list getAccountlist(){
return [Select id, Name,Industry,Phone from Account Order by CreatedDate desc limit 10];
}
@AuraEnabled
public static void getAccountupdatedlist(Account newAcc){
insert newAcc ;
}
}
[/sourcecode]
Next Step is to get the Lightning design system.You can download the latest version from https://www.lightningdesignsystem.com/resources/downloads and upload it as a static resource.
Now, first lets create a reusuable Lightning component so that we can use svg tags to show images.You can follow salesforce trailhead to create this. (https://developer.salesforce.com/trailhead/en/project/slds-lightning-components-workshop/slds-lc-4)
svg.cmp
[sourcecode language=”java”]
[/sourcecode]
svgRenderer.js
[sourcecode language=”java”]
({
render: function(component, helper) {
//grab attributes from the component markup
var classname = component.get(“v.class”);
var xlinkhref = component.get(“v.xlinkHref”);
var ariaHidden = component.get(“v.ariaHidden”);
//return an svg element w/ the attributes
var svg = document.createElementNS(“http://www.w3.org/2000/svg”, “svg”);
svg.setAttribute(‘class’, classname);
svg.setAttribute(‘aria-hidden’, ariaHidden);
svg.innerHTML = ”;
return svg;
}
})
[/sourcecode]
The next step will be to create a component to show the list of Accounts. We can use the reusuable svg component to add any images to the component by just passing the class and xlinkHref attribute in the component.
AccountListComponent.cmp
[sourcecode language=”java”]
Account NameIndustryPhone
{!acc.Name}{!acc.Industry}{!acc.Phone}
[/sourcecode]
The controller of the component calls an aura method and sets the accountlist attribute which is being used to show the accounts.
AccountListComponentController.js
[sourcecode language=”java”]
({
getAccountlst : function(component, event, helper) {
var action = component.get(“c.getAccountlist”);
action.setCallback(this, function(a) {
if (a.getState() === “SUCCESS”) {
component.set(“v.accountlist”, a.getReturnValue());
} else if (a.getState() === “ERROR”) {
$A.log(“Errors”, a.getError());
}
});
$A.enqueueAction(action);
}
})
[/sourcecode]
This is a very basic component which only shows the last 10 created accounts.Lets create a header component.
AccountListHeader.cmp
[sourcecode language=”java”]
Accounts
Add Account
10 items, ordered by recently created
[/sourcecode]
The controller of component is used for the new Account button which is adding a style to the modal dialog box(explained below).
AccountListHeaderController.js
[sourcecode language=”java”]
({
showModal : function(component, event, helper) {
document.getElementById(“backGroundSectionId”).style.display = “block”;
document.getElementById(“newAccountSectionId”).style.display = “block”;
}
})
[/sourcecode]
The next step is to create the modal dialog lightning component.By default I have added a style(diplay :none;) to the div so that it’s hidden by default.On the click of new Account button it calls a javascript button which changes the style of the div.
AccountCreateComponent.cmp
[sourcecode language=”java”]
[/sourcecode]
The controller of the component consists of two methods.One calls an aura method
to save the new account record.The other to hide the modal dialog by update the display style attribute.
AccountCreateComponentController.js
[sourcecode language=”java”]
({
showModalBox : function(component, event, helper) {
document.getElementById(“backGroundSectionId”).style.display = “none”;
document.getElementById(“newAccountSectionId”).style.display = “none”;
},
saveAccount : function(component, event, helper) {
var action = component.get(“c.getAccountupdatedlist”);
action.setParams({ “newAcc” : component.get(“v.newAccount”)});
action.setCallback(this, function(a) {
if (a.getState() === “SUCCESS”) {
document.getElementById(“backGroundSectionId”).style.display = “none”;
document.getElementById(“newAccountSectionId”).style.display = “none”;
} else if (a.getState() === “ERROR”) {
$A.log(“Errors”, a.getError());
}
});
$A.enqueueAction(action);
}
})
[/sourcecode]
Almost there, the last step is to combine all these 3 components 🙂
AccountListApp.app
[sourcecode language=”java”]
[/sourcecode]
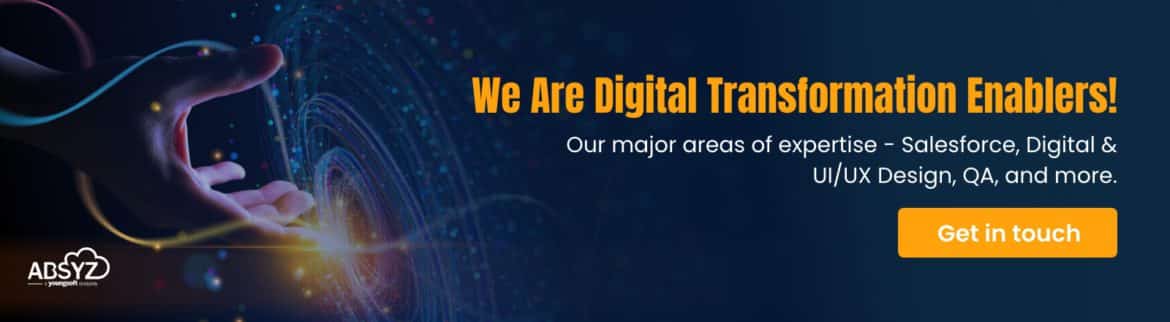